As suggested by some of our readers ,Today we are presenting new post for Ionic-2 with geolocation using angular.js
In this post for a sample we are going to build a weather app using the Forecast API by fetching current location.
Things we are going to do today is building a complete ionic app with side-menu, fetching data from API,asking for user location and much more..So,Let get Started!
Setting up and Creating a new Blank Project in Ionic:
Now let's create an app. From the terminal, go to your project folder. Type the following command to create a blank app with tabs.
Now let's create an app. From the terminal, go to your project folder. Type the following command to create a blank app with tabs.
Lets install ionic and cordova first,
npm install -g ionic cordova
Ionic framework now got installed on your machine,now lets create new blank project.
ionic start weatherapp blank
ionic build ios/Android
ionic emulate ios/android
Simply replace tabs in the above line with tab or side menu to create a tab app, or an application with a side menu.
Finally navigate to the project directory and add iOS and/or Android platforms to Ionic and test the app.
cd weatherapp
ionic platform add ios/Androidionic build ios/Android
ionic emulate ios/android
To complete the app we need to:
- Define the front end views
- Define controllers that provide data
- Define routes to tie the views to the controllers
Since we created a blank apps ,create a template folder and Navigate to the folder www/templates from your application root directory. This folder contains template files that will be rendered for the app.
Creating template files which we will use for Side-menu and fetching and showing weather UI using Forecast APIA)Create new template/menus.html file and paste the below code to it.
This file contains the html code for creating side-menus in your ionic applications.
We are try to fetch and convert the temperature either to Celsius/Fahrenheit using the ionic-radio component.
This file contains the html code for creating side-menus in your ionic applications.
We are try to fetch and convert the temperature either to Celsius/Fahrenheit using the ionic-radio component.
<ion-side-menus>
<ion-side-menu-content>
<ion-nav-bar class="bar-stable">
<ion-nav-buttons side="right">
<button class="button button-clear ion-navicon" menu-toggle="right"></button>
</ion-nav-buttons>
</ion-nav-bar>
<ion-nav-view name="menuContent">
</ion-nav-view>
</ion-side-menu-content>
<ion-side-menu side="right">
<ion-header-bar class="bar-stable">
<h1 class="title">Settings</h1>
</ion-header-bar>
<ion-content>
<ion-item class="item-divider icon ion-home"> Home</ion-item>
<ion-radio ng-model="settings.units" ng-value="'us'" menu-close="true" >Fahrenheit</ion-radio>
<ion-radio ng-model="settings.units" ng-value="'si'" menu-close="true" >Celsius</ion-radio>
<ion-item class="item-divider ">Cities</ion-item>
<ion-item class="item-divider">Location</ion-item>
</ion-content>
</ion-side-menu>
</ion-side-menus>
Next ,create the weather.html template which will fetch the weather details from Forecast API and showcase in UI.
The weather.html has the function of pull-to-refresh functionality which will be used to refresh the location.
The weather.html has the function of pull-to-refresh functionality which will be used to refresh the location.
<ion-view id="weatherContainer">
<ion-content>
<ion-refresher pulling-text="Pull to refresh..." on-refresh ="doRefresh()">
</ion-refresher>
<div id="current-weather" ng-show="haveData">
<h1 class="current-temp">{{currentTemp}}°</h1>
<h2>{{current.summary}}</h2>
<h3>
<span id="temp-hi"><i class="icon ion-arrow-up-c"></i>{{highTemp}}°</span>
<span id="temp-lo"><i class="icon ion-arrow-down-c"></i>{{lowTemp}}°
</span>
</h3> <h3>
<span id="temp-hi"><i class="icon ion-android-pin"></i>{{longi}}°</span>
<span id="temp-lo"><i class="icon ion-android-pin"></i>{{lati}}°
</span>
</h3>
</div>
</ion-content>
</ion-view>
Adding the template and main controller to index.html
Include the controller file and template file in the main index.hml to bind all the things at one.by creating three controller Navigate to js folder and create three js files.controller.js -handling Geolocation of the app by contacting the ngCordova plugin.app.js-Defining the routers and controller mappingservice.js-Sending the request to forecast API and updating with response.
Here Comes the Controller!
Important Methods while working the geolocation:
getCurrentPosition(options)
timeout -Maximum length of time (milliseconds) that is allowed to pass
maximumAge-Accept a cached position whose age is no greater than the specified time in milliseconds
enableHighAccuracy-Provides a hint that the application needs the best possible results
Returns Object with user information, such as id, lastName
watchPosition(options)
timeout-Maximum length of time (milliseconds) that is allowed to pass
maximumAge-Accept a cached position whose age is no greater than the specified time in milliseconds
enableHighAccuracy-Provides a hint that the application needs the best possible results
Returns Object - watchID which is used to clear watch later on.
clearWatch(watchID)
watchID- Uses the watchID returned from watchPosition()
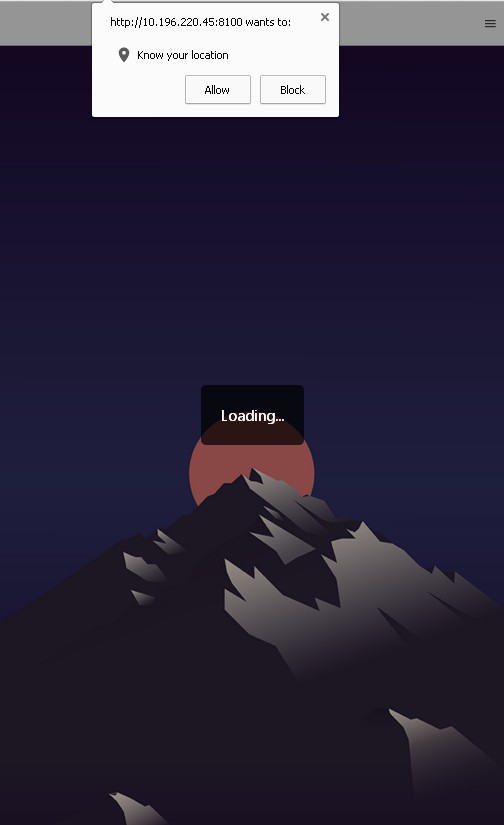
Include the controller file and template file in the main index.hml to bind all the things at one.by creating three controller Navigate to js folder and create three js files.controller.js -handling Geolocation of the app by contacting the ngCordova plugin.app.js-Defining the routers and controller mappingservice.js-Sending the request to forecast API and updating with response.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="initial-scale=1, maximum-scale=1, user-scalable=no, width=device-width">
<title></title>
<link href="lib/ionic/css/ionic.css" rel="stylesheet">
<link href="css/style.css" rel="stylesheet">
<!-- IF using Sass (run gulp sass first), then uncomment below and remove the CSS includes above
<link href="css/ionic.app.css" rel="stylesheet">
-->
<!-- ionic/angularjs js -->
<script src="lib/ionic/js/ionic.bundle.js"></script>
<script src="lib/ngCordova/dist/ng-cordova.min.js"></script>
<!-- cordova script (this will be a 404 during development) -->
<script src="cordova.js"></script>
<!-- your app's js -->
<script src="js/controllers.js"></script>
<script src="js/services.js"></script>
<script src="js/app.js"></script>
</head>
<body ng-app="app">
<ion-nav-view>
</ion-nav-view>
</body>
</html>
Here Comes the Controller!
Building the controller with angular.js that goes to the backend and does all the work
Navigate to js folder and add the below code to it.
angular.module('app', ['ionic', 'ngCordova', 'app.controllers'])
.constant('FORECASTIO_KEY', 'YOUR-API_KEY')//add your key here.
.config(function ($stateProvider, $urlRouterProvider) {
$stateProvider
.state('app', {
url: '/app',
abstract: true,
templateUrl: 'templates/menu.html',
controller: 'AppCtrl'
})
.state('app.weather', {
url: '/weather',
views: {
'menuContent': {
templateUrl: 'templates/weather.html',
controller: 'WeatherCtrl'
}
}
});
// if none of the above states are matched, use this as the fallback
$urlRouterProvider.otherwise('/app/weather');
})
.run(function($ionicPlatform) {
$ionicPlatform.ready(function() {
// Hide the accessory bar by default (remove this to show the accessory bar above the keyboard
// for form inputs)
if(window.cordova && window.cordova.plugins.Keyboard) {
cordova.plugins.Keyboard.hideKeyboardAccessoryBar(true);
}
if(window.StatusBar) {
StatusBar.styleDefault();
}
});
})
Creating the Controller.js which will be used to locate used using cordova geolocation and fetch the data from Forecast API.
getCurrentPosition(options)
timeout -Maximum length of time (milliseconds) that is allowed to pass
maximumAge-Accept a cached position whose age is no greater than the specified time in milliseconds
enableHighAccuracy-Provides a hint that the application needs the best possible results
Returns Object with user information, such as id, lastName
watchPosition(options)
timeout-Maximum length of time (milliseconds) that is allowed to pass
maximumAge-Accept a cached position whose age is no greater than the specified time in milliseconds
enableHighAccuracy-Provides a hint that the application needs the best possible results
Returns Object - watchID which is used to clear watch later on.
clearWatch(watchID)
watchID- Uses the watchID returned from watchPosition()
angular.module('app.controllers', ['app.services'])
.controller('AppCtrl', function ($scope, $log, Settings) {
$log.info('AppCtrl Created');
$scope.settings = Settings;
})
.controller('WeatherCtrl', function ($scope, $log, $ionicLoading, $ionicPlatform, $cordovaGeolocation, Location, Weather, Settings) {
$log.info('WeatherCtrl Created');
$ionicPlatform.ready(function () {
if (Location.lat == 0) {
var posOptions = {
timeout: 10000,
enableHighAccuracy: false
};
$cordovaGeolocation
.getCurrentPosition(posOptions)
.then(function (position) {
Location.lat = position.coords.latitude;
Location.long = position.coords.longitude;
getWeather();
}, function (err) {
// error
});
}
});
$scope.haveData = false;
$ionicLoading.show({
template: 'Loading...'
});
function getWeather() {
$scope.haveData = false;
$ionicLoading.show({
template: 'Loading...'
});
Weather.getWeatherAtLocation(Location.lat, Location.long).then(function (resp) {
$log.info(resp);
$scope.longi=resp.data.longitude;
$scope.lati=resp.data.latitude;
$scope.current = resp.data.currently;
$scope.highTemp = Math.ceil(resp.data.daily.data[0].temperatureMax);
$scope.lowTemp = Math.floor(resp.data.daily.data[0].temperatureMin);
$scope.currentTemp = Math.ceil($scope.current.temperature);
$scope.haveData = true;
$ionicLoading.hide();
$scope.$broadcast('scroll.refreshComplete');
}, function (error) {
alert('Unable to get current conditions');
$log.error(error);
});
}
//getWeather();
$scope.doRefresh = function () {
getWeather();
}
$scope.$watch(function () {
return Settings.units
}, function (newVal, oldVal) {
if (newVal !== oldVal) {
getWeather();
}
});
});
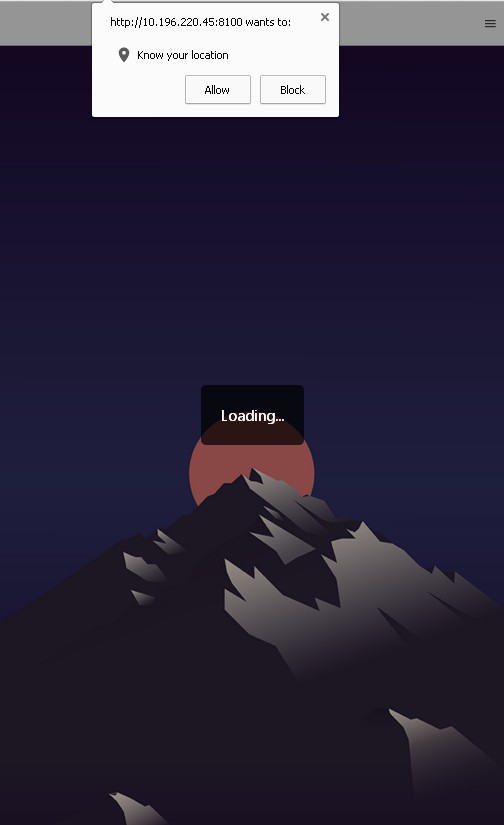
Setting Up and Calling the Forecast API through services.js
This will build the URL for forecast API with API key which we generated earlier and authenticate the URL to send request to API.
This will build the URL for forecast API with API key which we generated earlier and authenticate the URL to send request to API.
angular.module('app.services', [])
.factory('Settings', function () {
var Settings = {
units: 'us'
};
return Settings;
})
.factory('Location', function () {
var Location = {
lat: 0,
long: 0
};
return Location;
})
.factory('Weather', function($http, $log, Settings, FORECASTIO_KEY) {
$log.info('Weather Factory');
var url = 'https://api.forecast.io/forecast/' + FORECASTIO_KEY + '/';
return {
getWeatherAtLocation: function(lat, lng) {
return $http.jsonp(url + lat + ',' + lng + '?callback=JSON_CALLBACK&units='+Settings.units);
}
}
});
Run the ApplicationRun your application by below command. Ionic serve
EmoticonEmoticon