In the previous post, we got started with the python lesson which gave some basic idea about the syntax. We got started by writing a simple "Hello World program".In this lesson, we are going to get started with understanding variables in python. This lesson is again a beginner course to help you understand the basic concept. This post will cover different types of variables with syntax and deep dive into various type os variables like global & local variables.
What are the variables in python?
Variables are nothing but reserved memory locations to store values. This means that when you create a variable you reserve some space in memory.
Basic Syntax
Python variables do not need explicit declaration to reserve memory space. The declaration happens automatically when you assign a value to a variable. The equal sign (=) is used to assign values to variables.
Example:
Yes, that's the expected behavior. a, b and c are all set as labels for the same name. If you want three different value, you need to assign them individually.
In python, everything is an object, also "simple" variables types (int, float, etc..).
When you changes a variable value, you actually changes it's pointer, and if you compares between two variables it's compares their pointers. (To be clear, pointer is the address in physical computer memory where a variable is stored).
As a result, when you changes an inner variable value, you changes it's value in the memory and it's affects all the variables that point to this address.
For your example, when you do:
a = b = 5 This means that a and b points to the same address in memory that contains the value 5, but when you do:
a = 6 It's not affect b because a is now points to another memory location that contains 6 and b still points to the memory address that contains 5.
But, when you do:
a = b = [1,2,3] a and b, again, points to the same location but the difference is that if you change the one of the list values:
a[0] = 2 It's changes the value of the memory that a is points on, but a is still points to the same address as b, and as a result, b changes as well.
Example:
Local vs Global Variables in python
Global variables are the one that are defined and declared outside a function and we need to use them inside a function.
Consider the below example:
If a variable with same name is defined inside the scope of function as well then it will print the value given inside the function only and not the global value.
To make the above program work, we need to use “global” keyword. We only need to use global keyword in a function if we want to do assignments / change them. global is not needed for printing and accessing. Why? Python “assumes” that we want a local variable due to the assignment to s inside of f(), so the first print statement throws this error message("undefined: Error: local variable 'x' referenced before assignment"). Any variable which is changed or created inside of a function is local, if it hasn’t been declared as a global variable. To tell Python, that we want to use the global variable, we have to use the keyword “global”, as can be seen in the following example:
We have created a reference to global variable what if i want to delete and re-create a new.
How to delete every reference of an object in Python?
You can also delete the reference to a number object by using the del statement.
An object will be deleted as soon as all references to that object get removed. Python keeps a reference count internally - to illustrate :
The Python garbage collector will delete the object eventually. There is no guarantee in the CPython standard that the object will be deleted immediately, or at any time before the program ends - it depends how often the GC runs - how quickly your program runs, and whether there are circular references - (if two objects refer to each other, even if neither of the objects can be accessed through any names, the ref count of each object will never reach 0). Don’t rely on the ``__del__`` method to be triggered - it might be, but then again it might not.
Numbers(int)
Lists aren’t limited to a single dimension. Although most people can’t comprehend more than three or four dimensions. You can declare multiple dimensions by separating an with commas. In the following example, the MyTable variable is a two-dimensional array :
Tuple(tuple)
Tuple Vs List
Dictionaries can be more complex to understand, but they are great to store
data that is easy to access.
That’s pretty much it for this tutorial. I hope you enjoyed it!
I hope you can help me with more examples. I would also like to know if you
can share some example with usage in real algorithms with this data structures.
happy Coding !
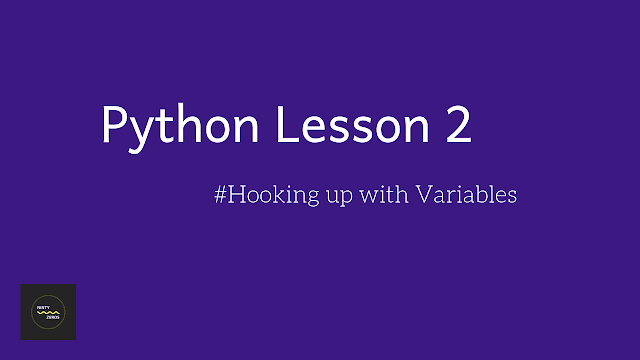
Prerequisite for this course
- A beginner
- python installed System (IDE/Interpreter).
- A notepad to take notes
What are actually variables?
In computer science, a variable is a value in a program that can change. It does not have to be a number — it can be a string (text value), a date, an amount of money, an object such as a picture, or simply null (which means it has no content). The value that is stored in a variable can change what happens when the program is run. Because of this, variables are commonly used to store input and output values.
What are the variables in python?
Variables are nothing but reserved memory locations to store values. This means that when you create a variable you reserve some space in memory.
Basic Syntax
Python variables do not need explicit declaration to reserve memory space. The declaration happens automatically when you assign a value to a variable. The equal sign (=) is used to assign values to variables.
Example:
count = 10 ### An integer value 10 assigned to count
name = "NintyZeros" ###A String value "NintyZeros" assigned to name
height = 2.8 ## A float type variables assiged
#Printing assigned variables
print("-"*30)
print("count :", count)
print("Name :", name)
print("Height :",height)
Can we do multiple assigment ?
Yes, that's the expected behavior. a, b and c are all set as labels for the same name. If you want three different value, you need to assign them individually.
In python, everything is an object, also "simple" variables types (int, float, etc..).
When you changes a variable value, you actually changes it's pointer, and if you compares between two variables it's compares their pointers. (To be clear, pointer is the address in physical computer memory where a variable is stored).
As a result, when you changes an inner variable value, you changes it's value in the memory and it's affects all the variables that point to this address.
For your example, when you do:
a = b = 5 This means that a and b points to the same address in memory that contains the value 5, but when you do:
a = 6 It's not affect b because a is now points to another memory location that contains 6 and b still points to the memory address that contains 5.
But, when you do:
a = b = [1,2,3] a and b, again, points to the same location but the difference is that if you change the one of the list values:
a[0] = 2 It's changes the value of the memory that a is points on, but a is still points to the same address as b, and as a result, b changes as well.
Example:
a=b=c=1; ###Yes,of course we can bro!
print("a:", a," b :" , b," c : " ,c)
-------------------------------------
a: 1 b : 1 c : 1
Local vs Global Variables in python
Global variables are the one that are defined and declared outside a function and we need to use them inside a function.
Consider the below example:
x = 0 ###Global Scope
def somefunction():
x=1 ####Local variable
print(x)
somefunction() ###X will be overwritten with local scope
print(x) ###Calling the Global scope value
#---------------------------
1 0
If a variable with same name is defined inside the scope of function as well then it will print the value given inside the function only and not the global value.
To make the above program work, we need to use “global” keyword. We only need to use global keyword in a function if we want to do assignments / change them. global is not needed for printing and accessing. Why? Python “assumes” that we want a local variable due to the assignment to s inside of f(), so the first print statement throws this error message("undefined: Error: local variable 'x' referenced before assignment"). Any variable which is changed or created inside of a function is local, if it hasn’t been declared as a global variable. To tell Python, that we want to use the global variable, we have to use the keyword “global”, as can be seen in the following example:
y = 0
def somefunction():
global y####global variable ####But not the great way to use it
y=1
print(y)
somefunction()
print(y)
-------------------------------
1 1
We have created a reference to global variable what if i want to delete and re-create a new.
How to delete every reference of an object in Python?
You can also delete the reference to a number object by using the del statement.
An object will be deleted as soon as all references to that object get removed. Python keeps a reference count internally - to illustrate :
del x,y
## Reference gets deleted
print(x)
#print(y)
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
<ipython-input-47-abdfb9bbb238> in <module>()
1 ## Reference gets deleted
----> 2 print(x)
NameError: name 'x' is not defined
Python Data Types
This guide is an overview of Python Data Types.
Overview
Python has five standard Data Types:
- Numbers(int)
- String(str)
- List(list)
- Tuple(tuple)
- Dictionary(dict)
Python sets the variable type based on the value that is assigned to it. Unlike more riggers languages, Python will change the variable type if the variable value is set to another value. For example:
Numbers(int)
var1 = 1
var2 = 10
type(var1)
Most of the time Python will do variable conversion automatically. You can also use Python conversion functions (int(), long(), float(), complex()) to convert data from one type to another. In addition, the
type
function returns information about how your data is stored within a variable.String(Str)
Create string variables by enclosing characters in quotes. Python uses single quotes
'
double quotes "
and triple quotes """
to denote literal strings. Only the triple quoted strings """
also will automatically continue across the end of line statement.
var1 = "Hello"
var2= "World"
print(var1+" "+var2)
type(var1)
--------------------------
Hello World
str
List(list)
Lists are a very useful variable type in Python. A list can contain a series of values. List variables are declared by using brackets
[ ]
following the variable name.Lists aren’t limited to a single dimension. Although most people can’t comprehend more than three or four dimensions. You can declare multiple dimensions by separating an with commas. In the following example, the MyTable variable is a two-dimensional array :
list = [ 'Ninty', 786 , 2.23, 'Zeros', 70.2 ] ### creating a list with mixed type of datatype
print(list) ###printing the content of the list
print(list[3]) ####Fetching values from index 3
print(list*2) ###Copying the values by 2
type(list) ###Checking the type of datatype
----------------------
['Ninty', 786, 2.23, 'Zeros', 70.2]
Zeros
['Ninty', 786, 2.23, 'Zeros', 70.2, 'Ninty', 786, 2.23, 'Zeros', 70.2]
Out[19]:
list
Tuple(tuple)
Tuples are a group of values like a list and are manipulated in similar ways. But, tuples are fixed in size once they are assigned. In Python the fixed size is considered immutable as compared to a list that is dynamic and mutable. Tuples are defined by parenthesis ().
tuple = ( 'Ninty', 786 , 2.23, 'Zeros', 70.2 ) ##Creating a tuple
print(tuple)
print(tuple[1])
print(type(tuple))
---------------------
('Ninty', 786, 2.23, 'Zeros', 70.2)
786
<class 'tuple'>
Tuple Vs List
Here are some advantages of tuples over lists:
- Elements to a tuple. Tuples have no append or extend method.
- Elements cannot be removed from a tuple.
- You can find elements in a tuple, since this doesn’t change the tuple.
- You can also use the in operator to check if an element exists in the tuple.
- Tuples are faster than lists. If you’re defining a constant set of values and all you’re ever going to do with it is iterate through it, use a tuple instead of a list.
- It makes your code safer if you “write-protect” data that does not need to be changed.
Cons:
list[2] = 100000 ###updating the index
print(list)
tuple(2) = 100000 ### Tou cannot update the tuple value
--------------------
['Ninty', 786, 100000, 'Zeros', 70.2]
File "<ipython-input-23-423972f5a01d>", line 3
tuple(2) = 100000 ### Tou cannot update the tuple value
SyntaxError: can't assign to function call
Dictionary(Dict)
Dictionaries in Python are lists of
Key
:Value
pairs. This is a very powerful datatype to hold a lot of related information that can be associated through keys
. The main operation of a dictionary is to extract a value based on the key
name. Unlike lists, where index numbers are used, dictionaries allow the use of a key
to access its members. Dictionaries can also be used to sort, iterate and compare data.Dictionaries are created by using braces ({}) with pairs separated by a comma (,) and the key values associated with a colon(:). In Dictionaries the
Key
must be unique. Here is a quick example on how dictionaries might be used:
dict = {} ### creating a dict
dict['one'] = "This is one" ## Adding data to dict by key as str
dict[2] = "This is two" ## Adding data to dict by key as int
print(dict) ##printing the dict
type(dict) ##Print the type as dict
print(dict['one']) ##Fetching value based on key
print(dict[2])
print(dict.keys()) ##Printing all the keys
print(dict.values()) ##Printing all the values
-------------------------------
{'one': 'This is one', 2: 'This is two'}
This is one
This is two
dict_keys(['one', 2])
dict_values(['This is one', 'This is two'])
Dictionaries can be more complex to understand, but they are great to store
data that is easy to access.
That’s pretty much it for this tutorial. I hope you enjoyed it!
I hope you can help me with more examples. I would also like to know if you
can share some example with usage in real algorithms with this data structures.
happy Coding !
1 comments so far
Detailed post .Thanks !
EmoticonEmoticon